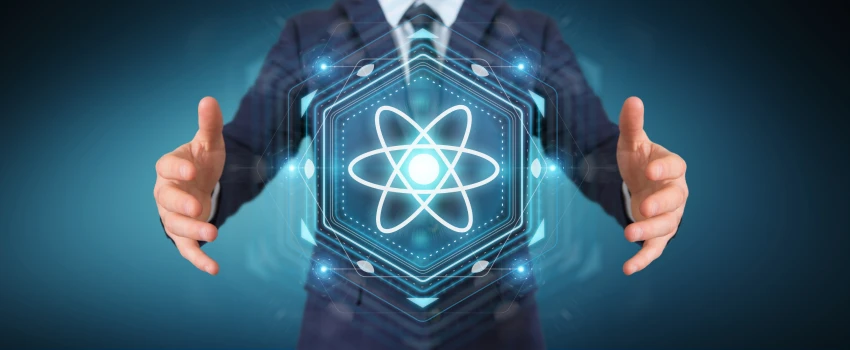
What’s React Server Component?
React Server Components is a new feature in React that allows developers to write components that can be rendered on the server and streamed directly to the client. With React Server Components, developers can build high-performance web applications that load faster and provide a better user experience.
Traditional server-side rendering in React involves sending the entire HTML page from the server to the client, which can be slow and inefficient. With React Server Components, only the components that need to be rendered are sent to the client, reducing the amount of data that needs to be transferred.
React Server Components also provide better support for real-time updates and collaborative editing. Since the components are rendered on the server, changes made by one user can be quickly reflected for other users, without requiring a full-page refresh.
One of the key benefits of React Server Components is that they are fully compatible with existing React code. Developers can easily integrate React Server Components into their existing React projects and start using them to build faster, more efficient web applications.
How React Server Components work:
React Server Components work by allowing developers to write components that can be rendered on the server and streamed directly to the client. Here is an overview of how React Server Components work:
- The client sends a request to the server for a specific page or component.
- The server receives the request and starts rendering the requested component.
- As the component is being rendered, React Server Components stream the markup to the client in small, incremental chunks.
- The client receives the markup as it is being streamed and starts rendering the component in the browser.
- Once the component is fully rendered on the server, it is “hydrated” on the client, which means that any interactivity and state management is activated.
- From this point on, the component behaves like a regular client-side React component, with the ability to respond to user interactions and update the UI in real-time.
React Server Components are designed to be highly efficient and performant, which means that they can help improve the speed and overall user experience of web applications. They are also highly flexible and can be integrated into existing React projects with minimal effort.
Read More: React Static Site Generation
Why use React Server Components:
- Improved performance: React Server Components can help improve the performance of your web application by reducing the amount of data that needs to be sent from the server to the client. By only streaming the components that need to be rendered, React Server Components can help reduce page load times and improve the overall user experience.
- Better collaboration: React Server Components are designed to support real-time updates and collaboration. Since the components are rendered on the server, changes made by one user can be quickly reflected for other users, without requiring a full page refresh.
- More flexible architecture: React Server Components are highly flexible and can be integrated into existing React projects with minimal effort. This makes them a good choice for developers who are looking to modernize their existing codebase without having to start from scratch.
- Improved SEO: Since React Server Components can be rendered on the server, they are more easily indexed by search engines, which can help improve the SEO of your web application.
- Better accessibility: React Server Components can help improve the accessibility of your web application by making it easier for screen readers and other assistive technologies to navigate and interact with your content.
Overall, React Server Components are a powerful new feature in React that can help improve the performance, collaboration, and overall user experience of your web application. If you’re building a modern web application and looking to take advantage of the latest technologies and best practices, React Server Components are definitely worth considering.
Using React Server Components:
- Install the experimental version of React that includes Server Components. This can be done using a package manager like npm or yarn.
- Set up your server to handle server-side rendering. This may involve installing additional dependencies, configuring your server to run Node.js, and writing code to handle rendering and serving your application.
- Define your Server Components using the React Server Component API. This involves defining a prepare function that returns the initial state of the component, as well as a render function that produces the output.
- Use your Server Components in your application code, just like you would use normal React components. However, keep in mind that Server Components may have some limitations, such as no access to browser APIs and limited interactivity.
- Render your application on the server using the ReactDOMServer API, and send the output to the client. This can be done using a framework like Next.js, or by writing your own server-side rendering code.
Example of React Server Component:
Here’s an example of a React server-side component that renders a simple greeting message:
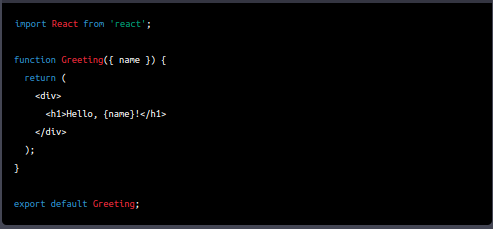
This component takes a prop called name and renders a greeting message with that name. You could use this component on the server to generate HTML that includes the greeting message, and then send that HTML to the client to be rendered in the browser.
To render this component on the server using Node.js and Express, you could do something like this:
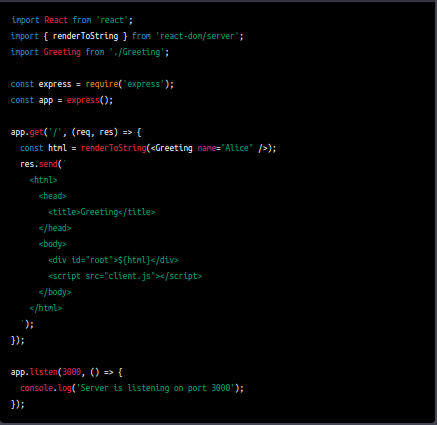
This code sets up an Express app that listens for requests on port 3000. When a request comes in for the root URL, the app renders the Greeting component to a string using renderToString, and then sends back an HTML page that includes that string. The client.js file could contain client-side React code that hydrates the server-rendered HTML and adds interactivity to the page.
What’s the benefit of React Server Component?
React Server Components offer several benefits that can help improve the performance, collaboration, and overall user experience of web applications. Here are some of the key benefits of React Server Components:
- Improved performance: React Server Components can help improve the performance of web applications by reducing the amount of data that needs to be sent from the server to the client. By only streaming the components that need to be rendered, React Server Components can help reduce page load times and improve the overall user experience.
- Better collaboration: React Server Components are designed to support real-time updates and collaboration. Since the components are rendered on the server, changes made by one user can be quickly reflected for other users, without requiring a full-page refresh.
- More flexible architecture: React Server Components are highly flexible and can be integrated into existing React projects with minimal effort. This makes them a good choice for developers who are looking to modernize their existing codebase without having to start from scratch.
- Improved SEO: Since React Server Components can be rendered on the server, they are more easily indexed by search engines, which can help improve the SEO of your web application.
- Better accessibility: React Server Components can help improve the accessibility of your web application by making it easier for screen readers and other assistive technologies to navigate and interact with your content.
Limitations of React Server Components:
- Experimental feature: React Server Components are still an experimental feature and are not yet available in the stable release of React. This means that they may be subject to breaking changes or other issues as they continue to be developed and refined.
- Requires server-side rendering: In order to use React Server Components, you need to have a server that can render the components and send them to the client. This can be more complex to set up and maintain than client-side rendering.
- Limited browser support: Since Server Components are still an experimental feature, they are not yet supported by all browsers. This can limit the number of users who are able to access your application.
- Learning curve: While Server Components are designed to be easy to use and integrate into existing React projects, there is still a learning curve involved. You may need to invest time in learning how to use Server Components effectively, especially if you are not already familiar with server-side rendering.
- Performance trade-offs: While Server Components can help improve performance in some cases, they may also introduce new performance trade-offs. For example, rendering components on the server may increase the load on your server, or require additional network requests to fetch data.
The main limitations set out by the spec, as compared with normal client-side components:
- No access to browser APIs: Since Server Components are rendered on the server, they have no access to browser APIs like window or document. This means that certain features or functionality that rely on browser APIs may not be possible to implement using Server Components.
- Limited interactivity: Server Components are designed to be stateless and deterministic, meaning that they should produce the same output given the same inputs. This can limit their ability to handle complex interactions or dynamic state changes that are common in client-side components.
- Limited third-party libraries support: Many third-party libraries and tools are designed specifically for client-side components and may not work as expected with Server Components. This can make it more difficult to find and use tools that are compatible with Server Components.
- Server-side rendering constraints: Server Components rely on server-side rendering to produce their output, which can be slower and more resource-intensive than client-side rendering. This can limit their scalability and performance in some cases.
Conclusion:
React Server Components are a new and experimental feature in React that aim to simplify server-side rendering and improve performance in certain scenarios. They offer many benefits over traditional server-side rendering, including faster time-to-interactive, improved SEO, and easier integration with existing React projects.
However, Server Components also come with some limitations, such as limited interactivity, no access to browser APIs, and constraints on server-side rendering. Additionally, since they are still an experimental feature, they may be subject to breaking changes or other issues as they continue to be developed and refined.
Overall, React Server Components show promise as a powerful tool for building high-performance, scalable web applications, but developers should carefully evaluate their suitability for their particular use case and consider the potential limitations before using them in production.
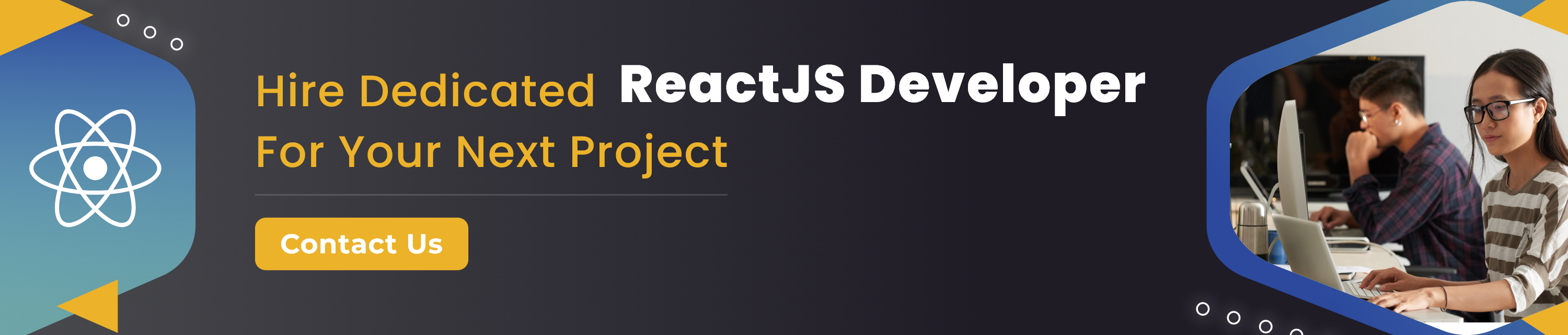